In Python programming, sets offer a versatile way to manage collections of unique elements. Adding elements to a set is a fundamental operation that Python simplifies with several methods tailored to different needs. Whether you’re adding single elements or merging multiple sets, understanding these methods empowers you to efficiently manipulate data structures. This article delves into various techniques for adding elements to sets in Python. We’ll explore essential methods such as add(), update(), and union(), each serving distinct purposes in set manipulation. From basic operations to advanced merging techniques, we’ll cover practical examples that demonstrate the flexibility and power of Python sets. By mastering these methods, you’ll not only learn how to expand and combine sets effectively but also discover best practices for managing sets in your Python projects. Whether you’re new to Python sets or looking to deepen your understanding, this guide will equip you with the knowledge to leverage sets efficiently in your programming endeavors.
Introduction to Python Sets
Python sets are versatile data structures designed to store collections of unique elements efficiently. Unlike lists or tuples, which allow duplicate elements, sets ensure each element is unique, making them ideal for tasks where uniqueness of elements is paramount.
A set in Python is defined by curly braces {} enclosing its elements, separated by commas. Here’s a basic example:

Key characteristics of Python sets include:
- Uniqueness: Sets automatically eliminate duplicate values when elements are added.
- Mutability: Sets are mutable, meaning you can add or remove elements after the set is created.
- Unordered Collection: Sets do not maintain the order of elements. The elements are stored in an arbitrary order.
Basics of Set Operations
Sets support a variety of operations for efficient data manipulation, including adding and removing elements, combining sets, and performing set operations such as intersection, union, difference, and symmetric difference. These operations make sets indispensable in scenarios requiring unique data handling, such as removing duplicates from a list, checking for membership, or performing mathematical set operations.
Basics of Set Operations
- Adding Elements to a Set:
Use the add() method to insert individual elements into a set, ensuring each element remains unique.
- Updating a Set:
The update() method allows you to add multiple elements from iterable objects like lists or other sets to an existing set.
- Merging Sets in Python:
Combine sets using the union() method to create a new set containing all unique elements from the original sets.
Using the add() Method
In Python, the add() method is used to insert a single element into a set. This method ensures that the element is added only if it does not already exist in the set, maintaining the set’s property of uniqueness. Here’s how you can use the add() method:
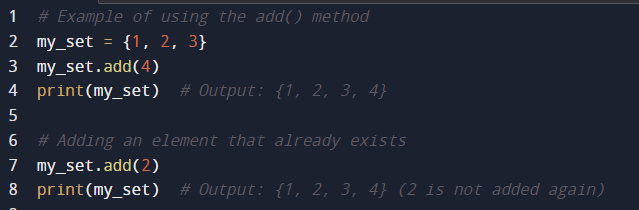
Practical Examples of Using add()
The add() method is particularly useful in scenarios where you want to dynamically add unique elements to a set. Here are a few practical examples:
- Filtering Unique Elements from a List:
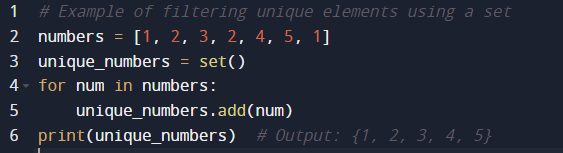
2. Tracking Unique Usernames:
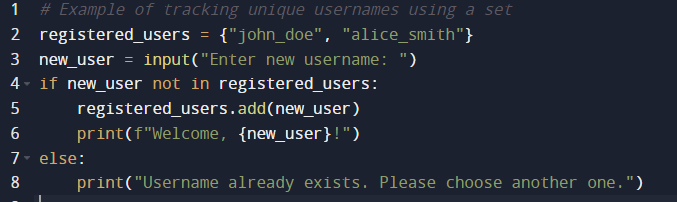
3. Counting Distinct Items:
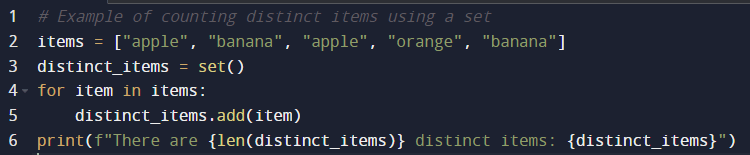
The add() method simplifies the task of managing unique elements in a set, ensuring efficient and effective data manipulation in Python.
Adding Multiple Elements with update()
In Python, the update() method is used to add multiple elements to a set from an iterable, such as a list or another set. This method ensures that duplicates are automatically eliminated, maintaining the set’s property of uniqueness. Here’s how you can use the update() method:
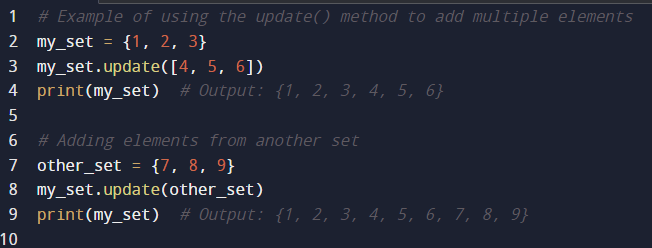
Merging Sets with union()
The union() method in Python is used to create a new set that contains all unique elements from two or more sets. Unlike update(), union() does not modify the original sets but instead returns a new set containing the merged elements. Here’s how you can use the union() method:
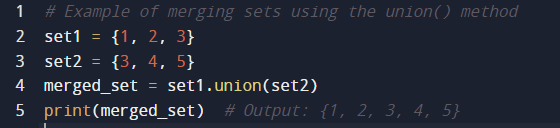
Combining Sets with update() and union()
In Python sets, the update() and union() methods serve distinct purposes despite both involving the combination of sets. The update() method modifies a set in place by adding elements from an iterable (such as another set or a list) while automatically handling duplicates. This method alters the original set directly, ensuring that it contains all unique elements from both the original set and the iterable.
On the other hand, the union() method does not modify the original sets but instead returns a new set that contains all unique elements from the original sets. It creates and returns a fresh set object without altering the original sets. This method is particularly useful when you want to merge sets temporarily for a specific operation without permanently changing the sets themselves.
In essence, update() is used for in-place modification of sets, directly adding elements to an existing set, while union() is used for creating a new set that combines elements from multiple sets without changing the original sets. Understanding these differences allows Python developers to choose the appropriate method based on whether they need to modify sets directly or create new sets for temporary or permanent use cases.
Combining update() and union() methods provides flexibility in manipulating sets in Python:
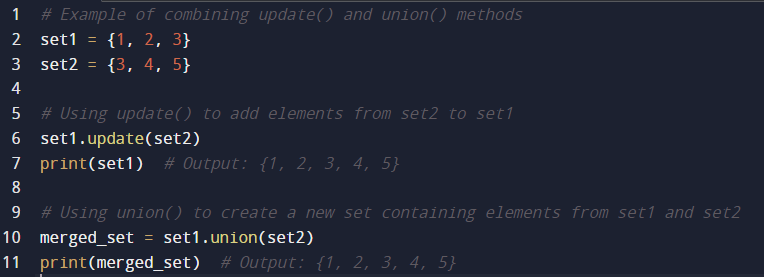
Best Practices for Managing Sets
When working with sets in Python, adhering to best practices ensures efficient and effective management of data. Here are some recommendations:
- Use Sets for Uniqueness: Leverage sets when you need to store unique elements. Sets automatically handle duplicates, ensuring data integrity without manual checks.
- Immutable Elements: Ensure elements stored in sets are immutable (e.g., integers, strings, tuples). Mutable types like lists or dictionaries cannot be hashed and therefore cannot be elements of a set.
- Understanding Set Operations: Familiarize yourself with essential set operations such as union, intersection, difference, and symmetric difference. These operations are efficient and performant for manipulating sets.
- Avoiding Direct Modification: Prefer using set methods like update() and union() for modifying sets rather than altering them directly with assignments or other methods.
- Clear and Concise Code: Write clear and concise code to manipulate sets. Python’s built-in set methods offer expressive and efficient ways to perform common operations.
Performance Considerations and Common Pitfalls
While sets provide efficient operations for unique data management, there are performance considerations and pitfalls to be aware of:
- Hashing Overhead: Set operations rely on hash tables for storing elements, which provide average-case constant-time complexity for lookups, inserts, and deletes. However, hashing involves computation overhead that can affect performance with large datasets.
- Memory Usage: Sets consume more memory than lists due to their internal hash table structure. Consider memory usage, especially when dealing with large sets or in memory-constrained environments.
- Mutable Elements: Avoid using mutable objects like lists or dictionaries as elements of a set, as their contents can change, potentially leading to unexpected behavior or errors.
- Performance of Operations: While set operations are generally efficient, the time complexity of operations like union or intersection can vary based on the size and contents of the sets involved. Be mindful of performance implications when working with large sets.
By following best practices and understanding potential pitfalls, Python developers can effectively manage and utilize sets for unique data handling while optimizing performance in their applications.
FAQ:
- How do you add elements to a set in Python?
In Python, elements can be added to a set using the add() method. This method takes a single argument, which is the element to be added to the set. If the element is already present in the set, the add() method does nothing, ensuring that sets maintain their property of containing only unique elements. For example, my_set.add(5) will add the element 5 to my_set if it’s not already there.
What is the difference between add() and update() methods?
add() method: This method adds a single element to a set. It takes one argument, which is the element to be added. If the element is already present in the set, add() has no effect.
update() method: Unlike add(), the update() method adds multiple elements to a set. It takes an iterable (like a list or another set) as an argument and adds each element from the iterable to the set. update() ensures that all elements remain unique within the set by handling duplicates automatically.
How can you merge two sets in Python?
Merging two sets in Python is straightforward using the union() method. This method returns a new set that contains all unique elements from both sets involved in the operation. It doesn’t modify either of the original sets but instead creates a new set with the combined elements. For instance, if you have two sets set1 and set2, set1.union(set2) will produce a new set containing all elements from both set1 and set2, ensuring each element is unique within the resulting set.