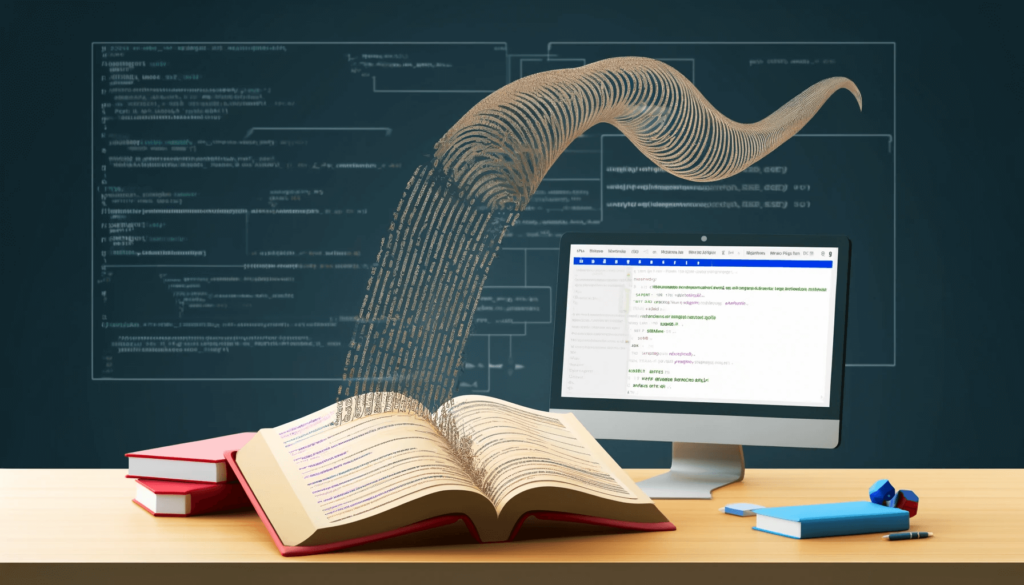
Reversing a string is a common task in programming, and Python offers multiple ways to achieve this. Whether you’re a beginner learning the basics of Python string manipulation or an experienced developer looking for efficient methods to reverse a sentence in Python, this comprehensive guide will walk you through various techniques and approaches. From using built-in Python string methods and string slicing to employing more advanced methods, we’ll explore a few Python reverse string techniques to help you master this fundamental programming skill. Additionally, we’ll delve into the Python reverse list function and showcase different ways for Python reverse string operations to suit your coding needs. By the end of this article, you’ll have a solid understanding of the different ways to reverse a string in Python and be equipped with the knowledge to implement these techniques in your own Python projects.
Introduction to String Reversal in Python
String reversal is a foundational operation in the realm of string manipulation and is frequently encountered in various programming tasks. Essentially, reversing a string involves rearranging its characters in the opposite order. For instance, reversing the string “Python” would result in “nohtyP”. This operation can be particularly useful in scenarios such as data processing, algorithmic challenges, and text manipulation tasks. Whether you’re dealing with palindrome detection, text encryption, or simply transforming data for display purposes, knowing how to reverse a string in Python is a fundamental skill that every developer should possess.
Using Slicing to Reverse Strings
String slicing is a powerful feature in Python that allows us to extract a portion of a string by specifying a start and end index. Utilizing slicing is one of the simplest and most straightforward ways to reverse a string in Python. The slicing syntax is intuitive and can be easily applied to achieve the desired result. Below, we’ll explore the slicing syntax and provide examples demonstrating how to reverse a string using this method..
Slicing Syntax and Examples
The general syntax for string slicing in Python is:
string[start:end:step]
- start: Starting index of the slice.
- end: Ending index of the slice.
- step: Step value for slicing (optional).
Examples:
1. Reverse a Single Word:
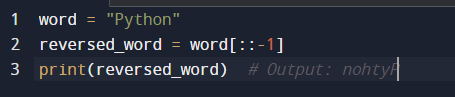
2. Reverse a Sentence

3. Reverse List of Strings:

Conclusion:
Using string slicing is one of the efficient ways to reverse a string in Python. Its simplicity and ease of use make it a preferred method for many developers. Whether you’re aiming to reverse a single word, a sentence, or a list of strings, string slicing, along with other python string methods, provides a versatile approach to achieve the desired outcome.
Advanced Methods: join() and reversed()
When it comes to more advanced and efficient ways to reverse a string or a list of strings in Python, the join() method combined with the reversed() function stands out. These methods not only offer a cleaner approach but also enhance the performance when dealing with larger strings or lists.
The join() Method in Action
The join() method is a string method that concatenates elements of an iterable, such as a list, into a single string. The reversed() function returns an iterator that accesses the given sequence in a reversed order. When combined, these methods provide an elegant solution to reverse strings or lists of strings.
Examples:
1. Reverse a Single Word:

2. Reverse a Sentence:
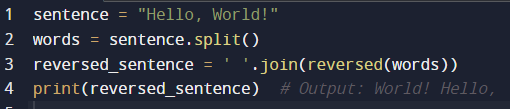
3. Reverse List of Strings:

Conclusion:
Utilizing the join() method along with the reversed() function provides a concise and efficient method to reverse a string or a list of strings in Python. This approach is versatile and can be applied to various scenarios, whether you want to reverse a single word, reverse a sentence in Python, or reverse list Python elements. With these advanced methods and other python string methods, you have multiple ways to achieve the desired string reversal effectively and elegantly.
Practical Applications: Reversing Sentences and Words
Understanding how to reverse sentences and words is not just an academic exercise; it has many practical applications in real-world programming scenarios. Whether you are working on text processing tasks, developing algorithms, or handling data transformations, the ability to reverse sentences and words can be incredibly useful. Below are some practical applications where reversing sentences and words can be beneficial:
Practical Applications:
- Text Analytics:
Language Model Training
Natural Language Processing Tasks
- Cryptography:
Encryption and Decryption Algorithms
- User Interface Design:
Text Animations
Dynamic and Interactive User Experiences
In this section, we’ll explore the step-by-step process of reversing a sentence and individual words within the sentence. We will delve into different methods to achieve this, including utilizing python using recursion reverse, the python reverse list function, and other versatile python string methods such as string slicing and the join() method. By understanding these techniques, you can enhance your proficiency in Python string manipulation and expand your toolkit for text processing and data transformation tasks.
Reversing a Sentence: Step-by-Step
Reversing a sentence in Python can be achieved through various methods, each with its own set of advantages and use-cases. Below, we’ll explore three different approaches to reverse a sentence, complete with detailed explanations and code examples.
1. Using String Slicing:

sentence[::-1]: Here, we use Python’s string slicing feature to reverse the entire sentence. The [::-1] slicing syntax reverses the string by stepping backward with a step of -1.
2. Using join() and reversed():

sentence.split(): This method splits the sentence into a list of words.
reversed(words): The reversed() function reverses the order of the words in the list.
‘ ‘.join(): Finally, the join() method concatenates the reversed words into a single string.
3. Using the Python Reverse List Function:
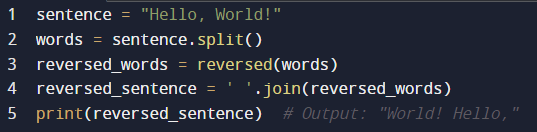
sentence.split(): This method splits the sentence into a list of words.
reversed(words): The reversed() function reverses the order of the words in the list.
‘ ‘.join(): Finally, the join() method concatenates the reversed words into a single string.
Key Difference:
The main difference between thejoin() and reversed() and the Python Reverse List Function lies in how the reversed list of words is handled:
- Method 2 (join() and reversed()) directly applies the reversed() function to the list of words within the join() method, without storing the reversed list in a new variable.
- Method 3 (Python Reverse List Function) first reverses the list of words using the reversed() function and then stores the reversed list in a new variable (reversed_words). This reversed list is then passed to the join() method to create the reversed sentence.
Both methods achieve the same result, but they differ in the way the reversed list of words is handled and utilized to form the reversed sentence.
Conclusion:
Reversing sentences and words is a valuable skill in Python programming with a wide range of practical applications. Whether you choose to use python using recursion reverse, the python reverse list function, or other python string methods like string slicing and join(), understanding the different ways to reverse a sentence in Python can greatly enhance your ability to handle text manipulation tasks effectively. With these techniques, you are well-equipped to tackle various scenarios requiring the reversal of sentences and words, making your code more versatile and robust.
FAQ:
- What is the easiest method to reverse a string in Python?
The easiest method to reverse a string in Python is by using string slicing. Python’s string slicing feature provides a simple and straightforward way to reverse a string without the need for additional functions or complex logic. By utilizing the slicing syntax [::-1], you can easily reverse the entire string in one line of code.
This method is straightforward and does not require any additional functions or operations. It is a direct and efficient approach to reverse a string in Python. While there are other ways to reverse a string, such as using the python using recursion reverse method, the python reverse list function, or various python string methods, the string slicing method stands out as the easiest and most intuitive way for many developers.
In comparison to other ways to reverse a string in python, like five python reverse string techniques or the reverse list python method, string slicing offers a concise and readable solution. It is a beginner-friendly approach that is widely adopted due to its simplicity and effectiveness. Thus, when looking for a straightforward and efficient way to reverse a string in Python, string slicing remains one of the most popular and easiest methods available.