JavaScript arrays are a fundamental data structure that developers frequently work with. As projects become more intricate, understanding and efficiently managing array differences become crucial. In this article, we will delve into various techniques for finding differences between arrays in JavaScript, ranging from basic to advanced methods.
Basic Techniques for Finding Differences Between Arrays
In JavaScript, comparing arrays and identifying differences can be accomplished through various techniques. One fundamental approach involves iterating through each element of the arrays and checking for disparities. This can be achieved using a loop, such as a for loop, to iterate over the elements and compare them individually.
Understanding the Role of Filter and Includes Methods
Alternatively, the Array.prototype.filter() method plays a crucial role in identifying distinctions between arrays. By employing this method, you can create a new array containing elements that meet specific criteria, allowing you to isolate the differing elements. Additionally, the Array.prototype.includes() method proves useful in determining whether a particular element is present in an array. Combining these techniques can provide a comprehensive strategy for efficiently finding differences between arrays in JavaScript.
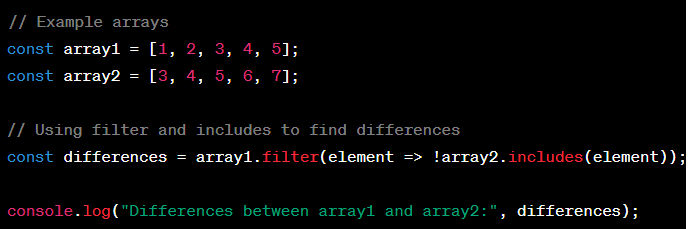
In this example, the filter method is employed to iterate over each element in array1. The callback function checks if the current element is not present in array2 using the includes method. Elements that satisfy this condition are retained in the differences array. The final output will be the elements that exist in array1 but not in array2. In this case, the output would be [1, 2], as these are the differences between the two arrays.
Using Set Objects to Identify Array Differences
The Set object in JavaScript provides another powerful way to identify array differences and explore symmetric differences. Here’s an example:
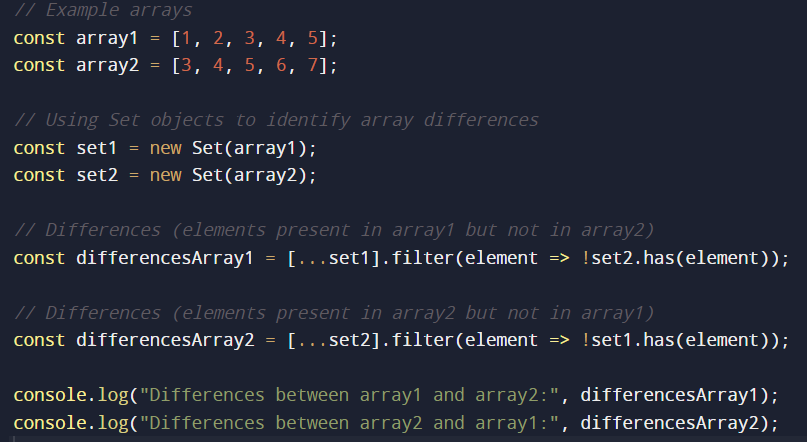
In this code snippet, two Set objects (set1 and set2) are created from array1 and array2. The spread operator ([…set]) is then used to convert the sets back into arrays. The filter method is applied to identify differences by checking whether elements are present in one set but not in the other.
Exploring Symmetric Differences in Arrays
The symmetric difference, in the context of sets or arrays, refers to the elements that are present in either of the sets but not in both. In other words, it represents the unique elements that distinguish each set.
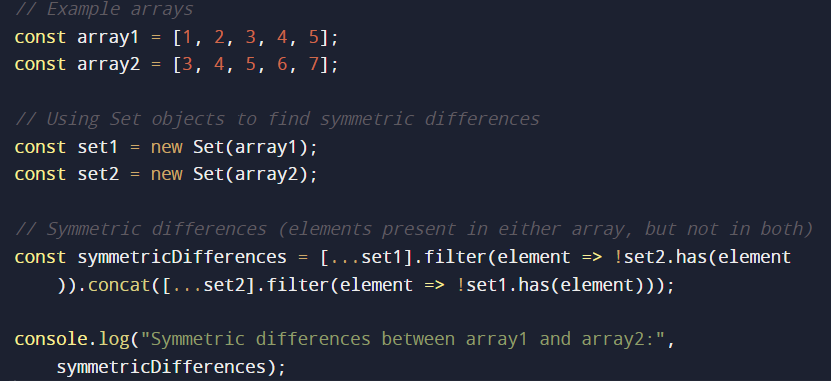
In this example, the symmetric differences between array1 and array2 are computed using Set objects, resulting in an array containing elements unique to each array, excluding those common to both arrays.
Implementing Array Difference with Lodash Library
Lodash is a popular utility library that offers numerous functions to streamline development and optimize code. One particularly useful function is _.difference, which simplifies the process of determining the variance between two arrays. The following example demonstrates the implementation of array differences using the Lodash library in JavaScript:
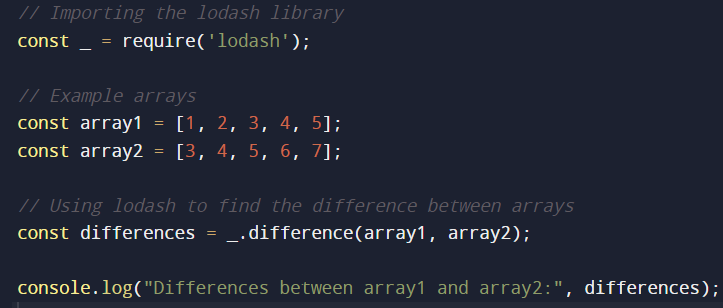
Practical Examples of JavaScript Array Differences
Now, let’s dive into practical examples to illustrate how these techniques can be applied in real-world scenarios, demonstrating their versatility and applicability in various use cases.
- Filter and Includes in Action:
Consider a scenario where you have two arrays representing user roles: currentRoles and newRoles. You want to find out which roles have been added to or removed from the user’s profile.
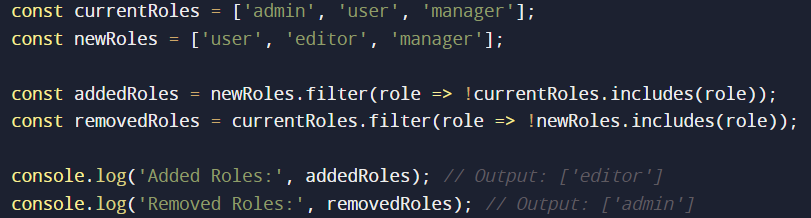
- Set Objects for Unique Elements:
Imagine you have an application where users can select their favorite genres, and you want to identify any changes made to their preferences. Set objects can simplify this comparison.
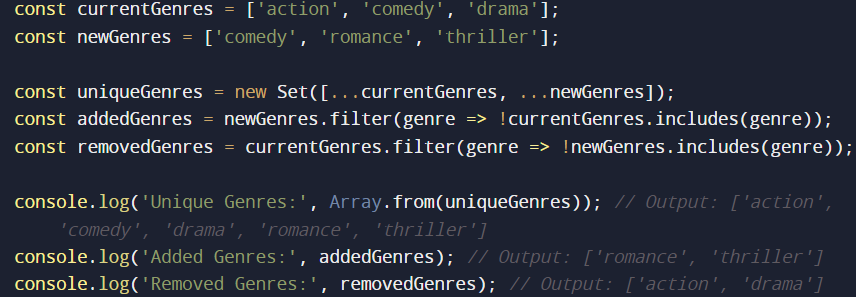
- Lodash for Simplified Array Difference:
Suppose you are working with an application that involves comparing large datasets, and you want to streamline the process using the Lodash library.
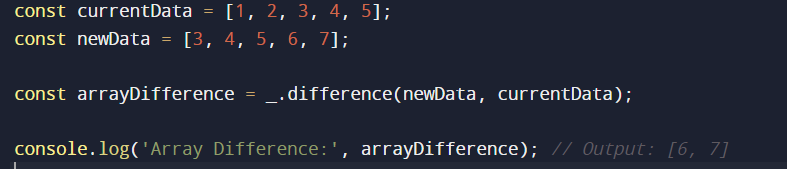
Best Practices for Efficient Array Comparison
Here, we’ll delve into best practices for optimizing array comparisons to ensure fast and resource-efficient operations.
- Utilize Set Objects for Uniqueness
- Optimize Search with Indexing (For large arrays, optimizing the search process can significantly improve performance. If the arrays are sorted, consider using binary search algorithms or maintaining indices to quickly locate elements)
- Use Map Objects for Enhanced Lookup
- Implement Parallel Processing for Large Datasets (When dealing with massive arrays, consider breaking down the comparison process into parallel tasks to leverage the full processing power of modern computers. Technologies such as Web Workers in the browser or the worker_threads module in Node.js allow for parallel execution without blocking the main thread.)
Advanced Methods for Complex Array Comparisons
When working with complex arrays in JavaScript, advanced methods become essential for accurate and efficient comparisons. However, it’s crucial to be aware of potential pitfalls that may arise in the process. Let’s explore advanced techniques first, followed by common pitfalls and strategies to avoid them.
- Custom Comparison Functions:
Advanced Use Case:
- Imagine you have arrays of objects with nested structures, and you need to compare them based on specific criteria within the nested properties.
Solution:
- Develop custom comparison functions tailored to your data structure. These functions should consider nested properties and any other complex structures within the objects
- Deep Equality Checks:
Advanced Use Case:
- Consider a scenario where arrays contain objects with deeply nested structures. A basic equality check might not capture differences within nested properties.
Solution:
- Implement a deep equality check that traverses nested structures to ensure a comprehensive comparison.
- Handling Arrays of Different Types:
Advanced Use Case:
- Arrays may contain a mix of data types, making direct comparisons challenging. For instance, one array might have numbers, and the other might have those numbers represented as strings.
Solution:
- Normalize the data types within the arrays during the comparison process. You can achieve this by converting elements to a common data type.
Common Pitfalls and How to Avoid Them
- Ignoring Asynchronous Operations:
Pitfall: Neglecting the asynchronous nature of data retrieval or processing within array comparison functions.
Avoidance: Utilize asynchronous patterns such as Promises or async/await. Ensure that the array comparison logic accommodates the asynchronous nature of the data being processed.
- Neglecting Edge Cases in Custom Comparison Functions:
Pitfall: Custom comparison functions may not account for all edge cases, leading to inaccurate results or unexpected behavior.
Avoidance: Thoroughly test custom comparison functions with various input scenarios, including edge cases and corner cases. Implement unit tests to ensure the reliability of the custom comparison logic.
- Inefficient Handling of Large Datasets:
Pitfall: Advanced methods may exhibit inefficiencies when applied to extremely large datasets, resulting in performance issues or crashes.
Avoidance: Implement strategies such as chunking, parallel processing, or pagination to handle large datasets more efficiently. Break down the array comparison into manageable portions to prevent resource exhaustion.
- Overlooking NaN and NaN Equality:
Pitfall: Comparisons involving NaN (Not a Number) can lead to unexpected outcomes due to the unique nature of NaN when compared using standard equality operators.
Avoidance: Use functions like isNaN or a custom equality check that considers NaN values if your arrays may contain them.
FAQ
What are the basic techniques for finding differences between two arrays in JavaScript?
In JavaScript, basic techniques for finding differences between two arrays include using native methods like filter and includes. The filter method allows you to create a new array containing elements that satisfy a specified condition, while includes checks for the presence of a particular element in an array. Another approach involves utilizing Set objects, which inherently store unique values, enabling straightforward identification of differences.
How can Set objects be used to identify differences in arrays?
Set objects in JavaScript provide a concise and efficient solution for identifying differences between arrays. By converting arrays into sets, developers can leverage set operations such as union, intersection, and difference. The Set data structure inherently ensures uniqueness, making it ideal for discerning elements unique to each array or those shared between them. The simplicity and elegance of Set operations streamline the process of array comparison.
What are the advantages of using the Lodash library for array comparison?
The Lodash library offers a dedicated difference function that significantly simplifies the process of finding discrepancies between arrays. One major advantage is its ease of use, providing a concise and readable syntax for array comparisons. Lodash also addresses potential pitfalls in native JavaScript methods, offering consistent behavior across different environments. Furthermore, Lodash is designed to optimize performance, making it particularly beneficial when dealing with large datasets or complex comparison scenarios.